Loops in Python
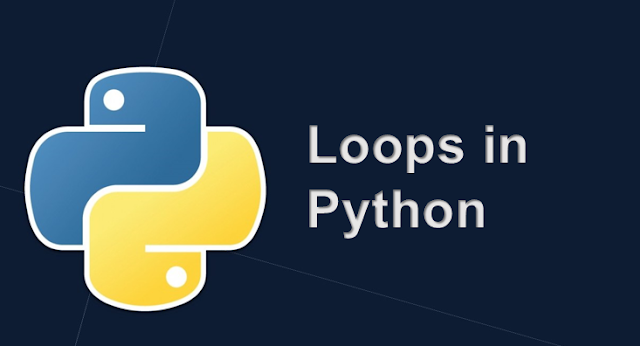
The Python programming language provides the following types of loops to handle looping requirements. Python offers three methods for executing loops. Although all methods offer the same basic functionality, they differ in their syntax and location check times.
Lets start
While loop:
In Python, loops are used to repeatedly execute a block of statements until a condition is satisfied. And when the situation is wrong, the line will run as soon as the loop in the program arrives.
Now we will see the Syntax
Syntax :
while (expression): statement(s)
All statements given the same number of character spaces after programming constructions are considered part of the same block code. Python uses indentation as a way to make its own statements.
Lets see this by an example
count
=
1 while
(count <
4
):
count
=
count
+
1
print
(
"Hello Dudle"
)
Output:
Hello Dudle
Hello Dudle
Hello Dudle
if
(condition):
# execute these statements in the block
else
:
# execute these statements in the block
while
(condition):
# execute these statements in the block
else
:
# execute these statements in the block
# combining else part with the while loop
count
=
1while
(count <
4
):
count
=
count
+
1
print
(
"Hello Dudle"
)
else
:
print
(
"comes to Else Block"
)
Hello Dudle
Hello Dudle
Hello Dudle
Comes to Else Block
While Block with single statement:
If the block contains the same statement as I did,
the whole loop can be declared in # while block with single statement
count
=
1while
(count
=
=
1):
print
(
"Hello Geek"
)
Note:
It is advisable not to use these types of loops, as this is an infinite loop
that never ends, where the situation is always correct and you must end the compiler.
See this for an example of using a loop for iterations. As mentioned in the article,
it is not recommended to use loops for iterations in Python.
For loops:
Used for row traversal for loops. For example: Detecting list or string
or array In Python, there is no C style for loops, i.e. (i = 0; i <n; i ++). The
loop contains "for", which is the same for each loop in other languages. Let's learn
how to use loop for sequential travels.
Lets see the Syntax:
for iterator_variable in range:
statements(s)
# Iterating over range 0 to n-1
n
=
5for
i
in
range
(
0
, n):
print
(i)
Output :
0
1
2
3
4
Lets see some other codes
# Iterating over a list for this
print
(
"List Iteration"
)
l
=
[
"code"
,
"for"
,
"dudle"
]
for
i
in
l:
print
(i)
# Iterating over a tuple (immutable)
print
(
"Tuple Iteration\n"
)
t
=
(
"code"
,
"for"
,
"dudle"
)
for
i
in
t:
print
(i)
# Iterating over a String
print
(
"String Iteration\n"
)
s
=
"code"
for
i
in
s :
print
(i)
# Iterating over dictionary
print
(
"Dictionary Iteration\n"
)
d
=
dict
()
d[
'xyz'
]
=
124d[
'abc'
]
=
344
for
i
in
d :
print
(
"%s %d"
%
(i, d[i]))
Output:
List Iteration
geeks
for
geeks
Tuple Iteration
geeks
for
geeks
String Iteration
c
o
d
e
Dictionary Iteration
xyz 124
abc 344
Iterating Changes by index of sequence: We can also use the index of elements in an order. The main idea is to first calculate the list length and then redirect it over the order of this length range.
See examples below:
# Iterating by index sequence
list
=
[
"code"
,
"dudle
"
]
for
index
in
range
(
len
(
list
)):
print
list
[index]
Output :
code
dudle
Using else statement with the for loops:
Other statements in the loop can also be included in the loop. Since there are no
conditions for the loop based on which execution ends, any other block will be executed after the block finishing execution.
The following examples show how to do this:
# combining else part with for looping
list
=
[
"code"
,
"dudle
"
]
for
index
in
range
(
len
(
list
)):
print
list
[index]
else
:
print
"Inside the Else Block"
Output:
code
dudle
Inside the Else block
We can use loop for user-defined iterations. See this for example.
Nested loops:
Python programming language allows to use one loop
inside another loop. The following concept shows some examples to
illustrate the concept.
Syntax:
for
iterator_variable
in
sequence:
for
iterator_variable
in
sequence:
statements(s)
statements(s)
while
expression:
while
expression:
statement(s)
statement(s)
The last note on the loop niche here is that we can place any type of
loop inside
Lets see by an example:
# nested for loops in Python
from
__future__
import
print_function
for
i
in
range
(
1
,
6
):
for
j
in
range
(i):
print
(i, end
=
' '
)
print
()
Output :
1
22
333
4444
55555
Loop Control Statement:
The loop control statement replaces the
execution from its normal sequence. When
Python supports the following control statements.
# Prints all letters except 'o' and 'u'
for letter in 'codedudle':
if letter == 'o' or letter == 'u':
continue
print 'Current Letters are :', letter
var = 8
Output
Current Letters are : c
Current Letters are : d
Current Letters are : e
Current Letters are : d
Current Letters are : d
Current Letters are : l
Current Letters are : e
Break Statement:
It brings the control out of the loop and stop the execution
for letter in 'codedudle':
if letter == 'o' or letter == 'u':
break
print 'Current Letter are:', letter
Output:
Current Letter are: o
Pass statement:
We use pass statement to write a blank loop. Pass space control is also used for announcements, functions and classes.
for letter in 'codedudle':
pass
print 'Last Letter are :', letter
Output :
Last Letter are : e
In this article i have explained the looping syntax in python.
Recommended :
- Techniques of Looping
- Operators in Python
- Ternary Operator with examples in Python
- Any and All in Python
- Inplace and Standard operator in python
- Python a += b is not always a = a + b
- Difference between == and is operator
- Python Membership and Identity Operators
0 Comments
If you have any doubt, Please let me know.